Basic Tutorials¶
Basic python example¶
1import json
2import requests
3
4
5# Set the root API url and the endpoint of your choice.
6# The list of endpoints is given under the "REST API" section.
7url = 'https://geodata.dri.edu/raster/mapid/values'
8
9# Add your token to the header (replace the <insert your api token> with your token.
10headers = {"Authorization": "<insert your api token>"}
11
12# Set your parameters
13params = {
14 'dataset': 'GRIDMET',
15 'variable': 'tmmx',
16 'temporal_statistic': 'mean',
17 'start_date': '2016-01-01',
18 'end_date': '2016-02-01'
19}
20
21# Make the call
22r = requests.get(url, params=params, headers=headers, verify=False)
23
24# Read the response
25print(json.dumps(r.json(), indent=2))
{
"mapid": "projects/earthengine-legacy/maps/63c8af524e2cca26fe476cae69b45775-3f43b995dd1e5edc151123e46d83f571",
"token": "",
"tile_fetcher": "https://earthengine.googleapis.com/v1alpha/projects/earthengine-legacy/maps/63c8af524e2cca26fe476cae69b45775-3f43b995dd1e5edc151123e46d83f571/tiles/{z}/{x}/{y}",
"mapid": "63c8af524e2cca26fe476cae69b45775",
"access_token": "3f43b995dd1e5edc151123e46d83f571",
"colormap_options": {
"min": -5,
"max": 35,
"palette": [
"#2166ac",
"#4393c3",
"#92c5de",
"#d1e5f0",
"#fddbc7",
"#f4a582",
"#d6604d",
"#b2182b"
],
"opacity": 0.7,
"colorbar_ticks": [
-5.0,
0.0,
5.0,
10.0,
15.0,
20.0,
25.0,
30.0,
35.0
]
}
}
Downloading files from Google Bucket¶
1import requests
2
3root_url = 'https://storage.googleapis.com/'
4bucket_name = '<your bucket name>/'
5folder = '<folder if present>/'
6filename = '<your filename>'
7
8url = root_url + bucket_name + folder + filename
9
10def download_file(url):
11 download_filename = url.split('/')[-1]
12
13 with requests.get(url, stream=True) as r:
14 r.raise_for_status()
15 with open(download_filename, 'wb') as f:
16 for chunk in r.iter_content(chunk_size=8192):
17 f.write(chunk)
18 return download_filename
19
20download_file(url)
Testing your map id¶
Sample code and instructions are located at https://github.com/Google-Drought/MapidTestServer.
The readme page will step through setting up a node.js test server (instructions are for Mac OS) to display your newly created urls on your local machine. See example image for an idea of what it will look like.
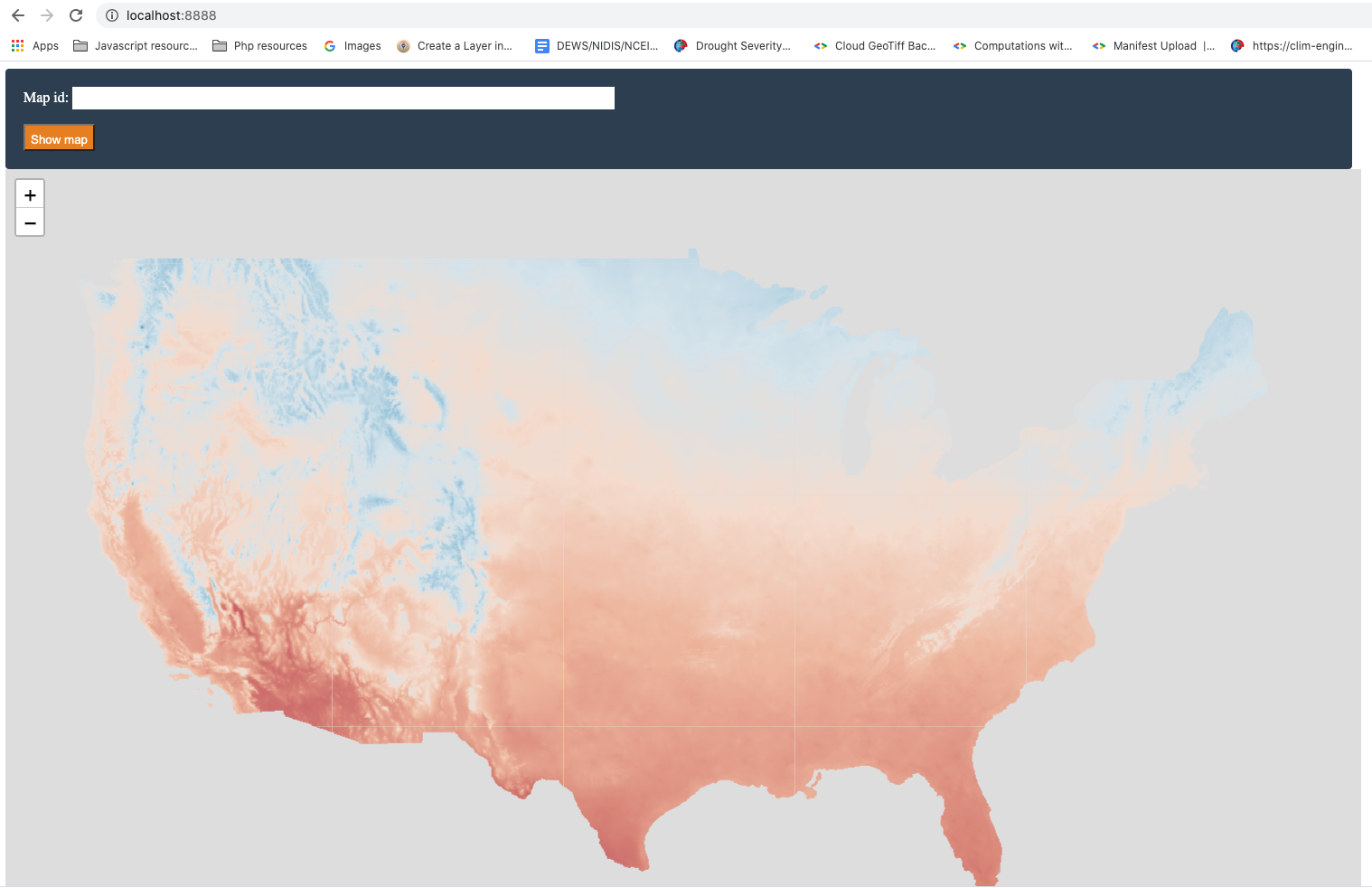